Class Projects
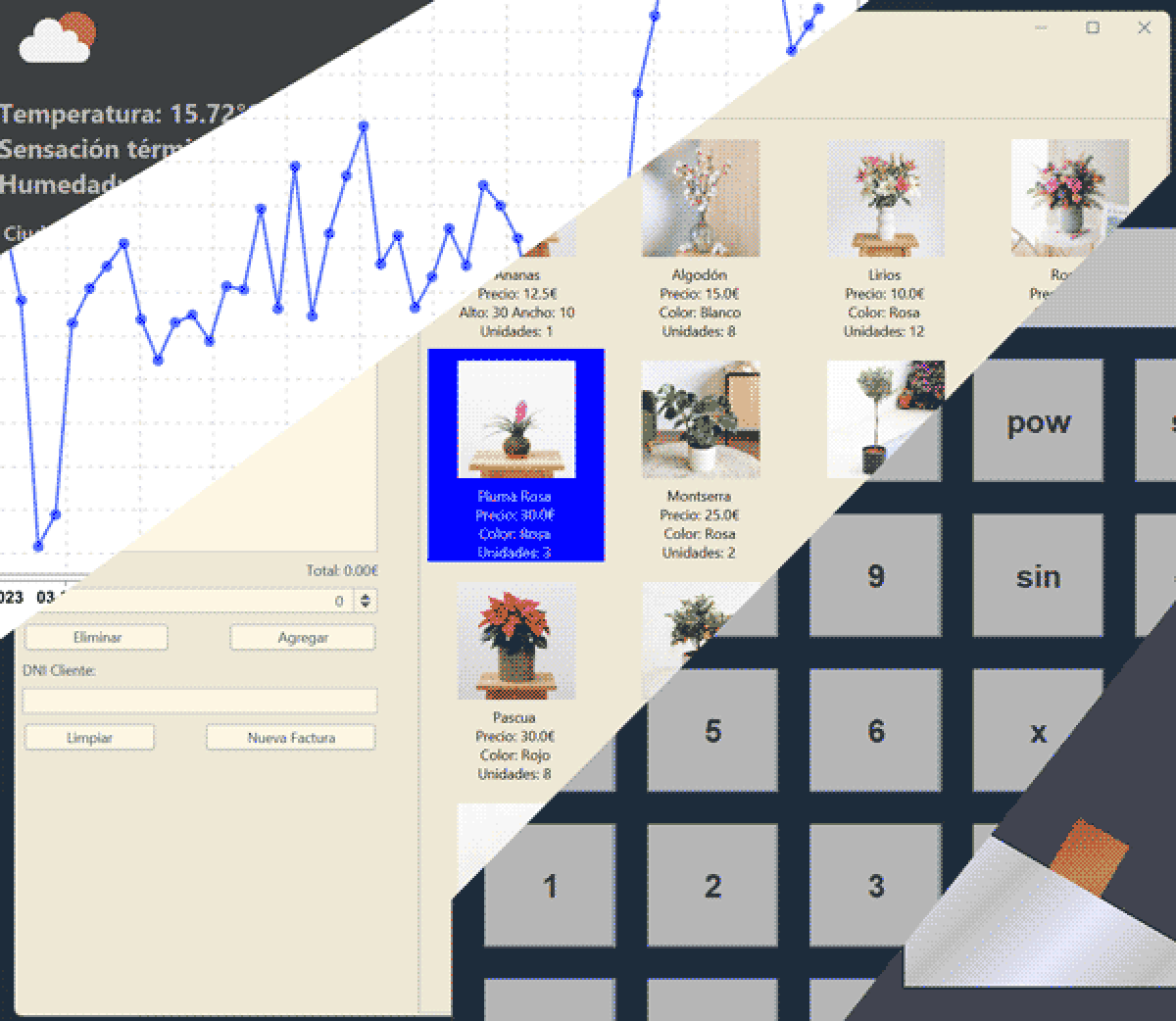
Foreword
The main purpose of these projects was to pass my professional training in multiplatform programming. Although I tried to do everything possible to deliver robust projects that met all the requirements, the pace at which we worked often forced me to seek compromises. In many cases, it was preferable to deliver on time even if not all the errors were resolved, so there are surely errors in many of the programs. On the other hand, although I have tried to choose projects that are exclusively mine, in some cases there is code from a colleague, Víctor Alocén, since it was mandatory to work in pairs. Thank you, Víctor. These two years would not have been so edifying if I had not had a colleague like you, who always showed me solutions that would not have occurred to me and who shared my desire to always give the best in each project with the idea of learning as much as possible.
Sports Center Path Finder
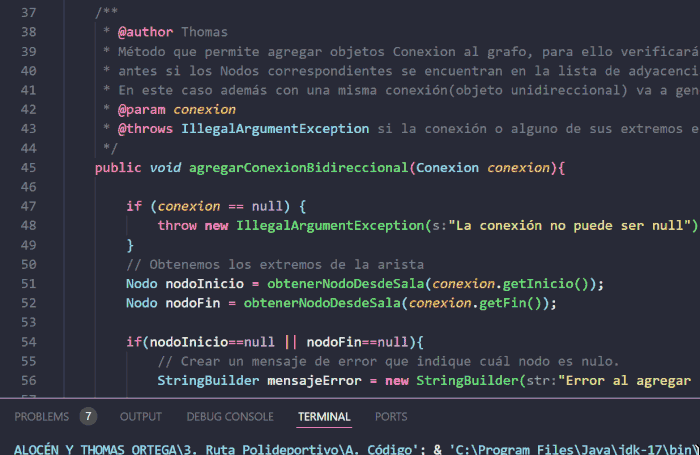
Project Overview
This is a Java application designed to find the shortest path between two locations in a sport complex. It implements a graph data structure and Dijkstra's algorithm to calculate optimal routes. To make it more engaging a GUI was added to visualize the sports center map and display the optimal route between two facilities.
Technical Highlights
- Graph Implementation: Custom graph structure using adjacency lists
- Dijkstra's Algorithm: Efficient pathfinding between nodes
- GUI Development: Java Swing for user interaction and path visualization
- Object-Oriented Design: Utilization of interfaces and inheritance for flexibility
- Error Handling: Robust exception management for edge cases
Key Features
- User selection of start and end locations
- Real-time calculation and display of the shortest path
- Distance calculation for the chosen route
- Extensible design for easy addition of new locations
Graph Design
While coupling between nodes and connections is implemented in the design, at the end it was easier to map the Graph using a HashMap instead of iterating in each object internally to find out in their properties how they are connected.
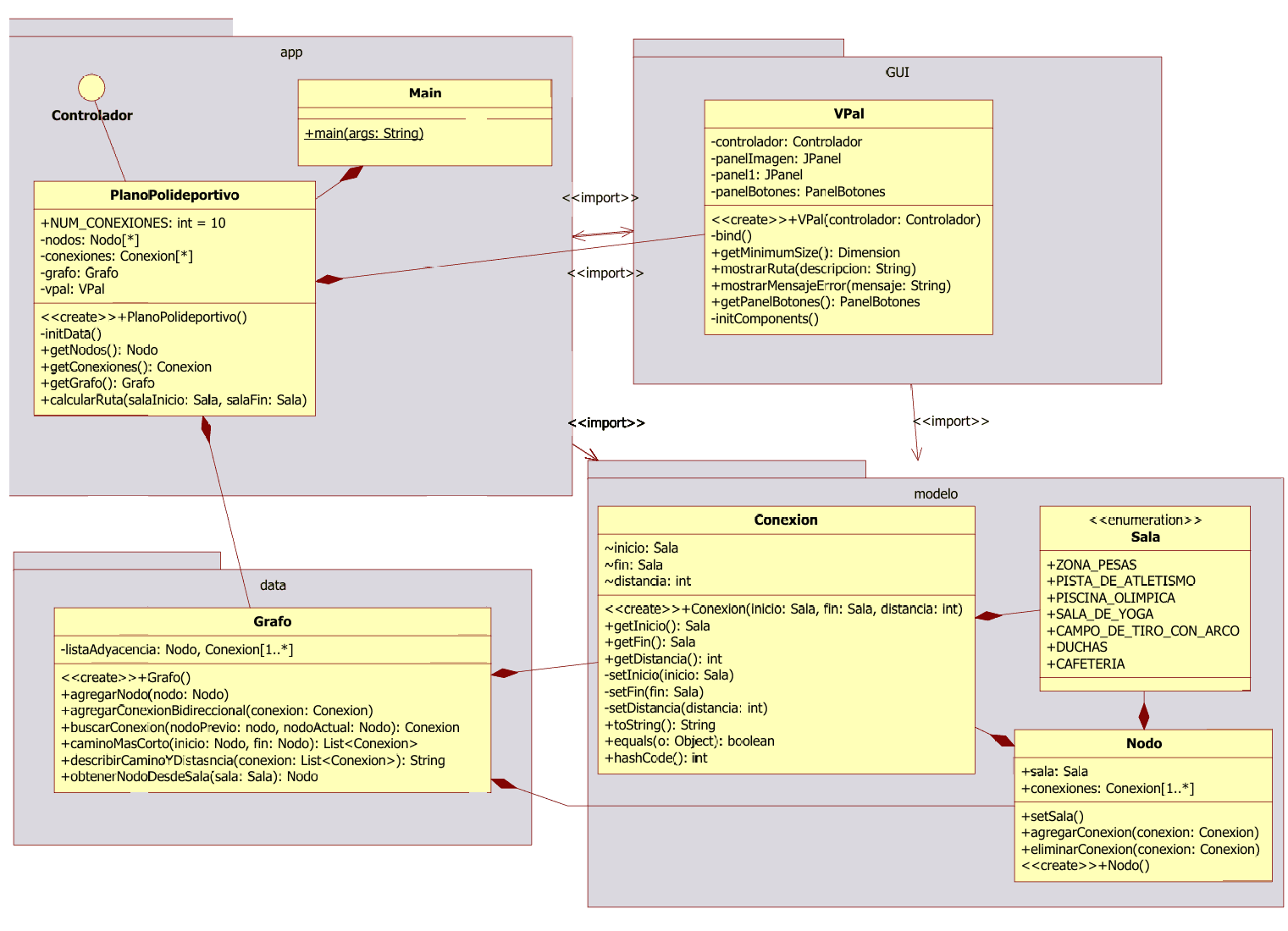
Setup for the algorithm
While I won't talk in detail about how to implement the steps of the algorithm, you can check my setup and overall steps to run it:
public ArrayList<Conexion> caminoMasCorto(Nodo inicio, Nodo fin) {
// Implementation of Dijkstra's algorithm
Set<Nodo> nodosVisitados = new HashSet<>();
Map<Nodo, Integer> distanciasMinimas = new HashMap<>();
Map<Nodo, Nodo> nodosPrevios = new HashMap<>();
// Initialize distances
for (Nodo nodo : listaAdyacencia.keySet()) {
distanciasMinimas.put(nodo, nodo.equals(inicio) ? 0 : Integer.MAX_VALUE);
nodosPrevios.put(nodo, null);
}
// Dijkstra's main loop
while (nodosVisitados.size() < listaAdyacencia.size()) {
// ... (algorithm implementation)
}
// Reconstruct path
ArrayList<Conexion> caminoMasCorto = new ArrayList<>();
// ... (path reconstruction)
return caminoMasCorto;
}
Challanges and Solutions
- Challenge: Represent the layout of the facility as a Graph. Solution: Implementad a Custom 'Node' and 'Connection' to represent locations and paths.
- Challenge: Making the app engaging for the user. Solution: Use of Java Swing to create the simpliest but still easy to use GUI.
Learning Outcomes
- It was the first time I worked with pathfinding algorithms, implementing the code after reviewing the theory was quite interesting.
- Improved my proficiency using data structures, OPP and MVC.
Flower Shop POS System
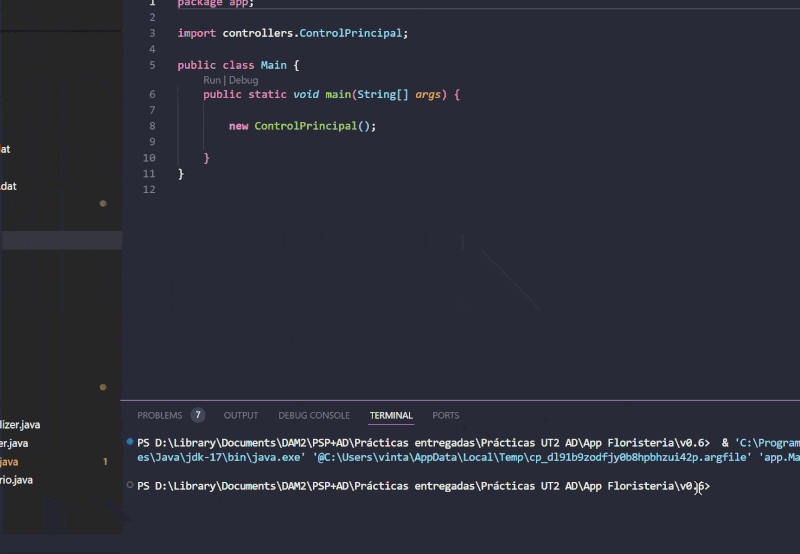
Project Overview
This Java application was designed to manage the inventory and sales of a little flower shop, some of the requeriments were the ability to manage items with different properties, persistence using files, the ability to manage inventory and sales(CRUD operations), the ability to check the inventory and sales of given day and some kind of user interface.
Technical Highlights
- Implemented persistence using binary files: Each subsystem (inventory and sales) has their own design and custom serialization.
- MVC Arquitecture: Follows a common design pattern to manage system design, user interactions and GUI.
- Custom GUI components: Developed a custom Swing component to display a grid of products using the observer pattern to ensure synchronization with the rest of the system.
- Object-Oriented Design: Utilization of interfaces and inheritance that allows a flexible transition between the inventory and sales system.
- Error Handling and Validation: Robust exception management and validation of data.
- Testing: Basic unit testing and integration tests were necessary to valide the systems and their integration.
Key Features
- Inventory Management: Tracks items with different attributes.
- Sales System: Procceses sales transactions and generates invoices (JSON).
- Persistence: Utilizes binary files for inventory and JSON for individual sales records.
- GUI: User-friendly interface built with Java Swing, JFormDesigner, featuring custom components.
Observer Pattern
A common pattern design that enables synchronization between the inventory and its visual representation, keep in mind that these classes come from different files of the project, I'm presenting them in the same block to have an easier time understanding how they work together:
// Defining the interface
public interface InventarioObserver {
void onInventarioChanged();
}
// Our inventory manager will have a list of observers
public class GestionInventario {
// The list is used to notify the observers when a change is made
private List<InventarioObserver> observers = new ArrayList<>();
// For example when adding items
public void agregarItem(InventarioItem item) throws IOException {
// rest of the method logic
notifyObservers();
}
// For this task we can use a simple method like this
private void notifyObservers() {
for (InventarioObserver observer : observers) {
observer.onInventarioChanged();
}
}
}
// Then our GUI classes would implement the interface
public class PanelGridProductos extends JPanel implements InventarioObserver {
@Override
public void onInventarioChanged() {
try {
// Method that holds the logic to refresh the items and their properties
recargarProductos();
} catch (IOException e) {
// Exception management logic
}
}
}
// Lastly, our controller has the task to initialize and register the observers
public class ControlPrincipal implements ActionListener {
// In our program we have the Grid and a table that tracks the items that will be sold
private void initObservers(){
gestorInventario.registerObserver((InventarioObserver)ventana.getPanelGridInventario());
gestorInventario.registerObserver((InventarioObserver)ventana.getPanelGridFactura());
}
}
Challanges and Solutions
- Inventory Synchronization: Implemented an observer pattern to ensure real-time updates across the application.
- GUI Design: Java Swing is an old library for GUI creation but it was the one that we were taught in class, implementing a modern component like a Grid was quite challenging and required a good understanding of the Swing library.
Learning Outcomes
- The scope of this project was a good test to see if I was making progress as a programmer, from low level operations like designing binary files, to more high level patterns like ones the mentioned above.
- This project required robust components that were possible because I gained familiarity with the language and libraries like Java Swing. Testing each system and their integration was crucial to achieve the desired behaviors.
Math Server
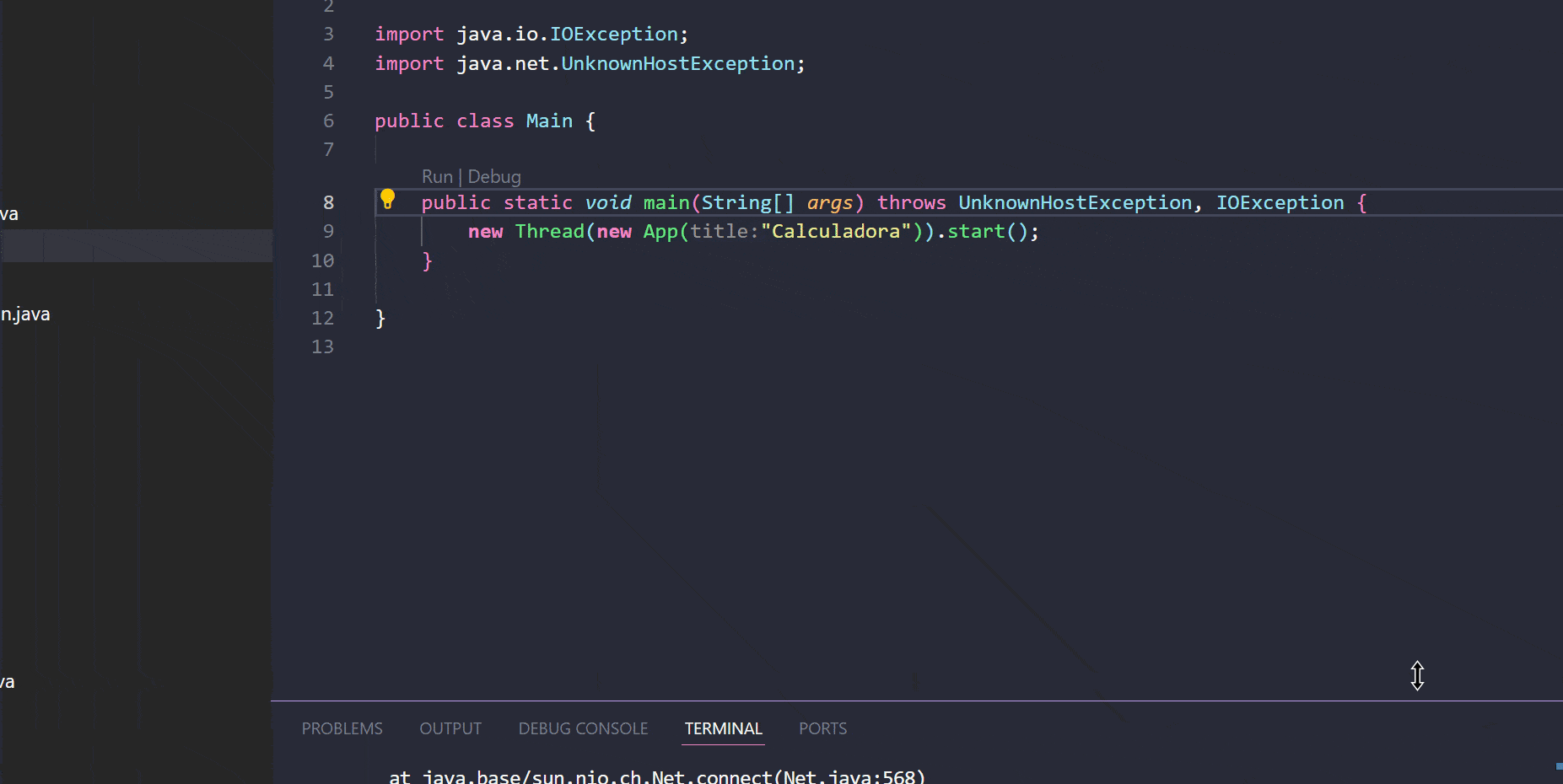
The Math Server is a Java-based client-server application able to perform various mathematical operations. Clients are able to send mathematical operations as queries to the server, which processes them and returns the results. The server supports basic operations as well as more complex functions like roots, powers and trigonometry.
Technical Highlights
- Client-Server Architecture: Implements a TCP/IP socket-based communication system.
- Multi-threading:Handles multiple client connections simultaneously.
- Protocol Design: Custom protocol for client-server communication.
- Cross-Platform Clients: Implemented in both Java (Swing) and C# (WPF .NET Core 8).
- JavaScript Integration: Uses Nashorn library to execute JavaScript for complex calculations.
Key Features
- Supports operations: +, -, *, /, Sine, Cosine, Roots, and Powers.
- Robust error handling for invalid operations or communication issues.
- Graphical user interface for clients, functioning as a calculator.
- Minimal server-side control panel for management (shutdown).
Solution Approach
For this project our priority was defining the steps to make the communication of clients and server possible, a sequence diagram was made for this purpose:
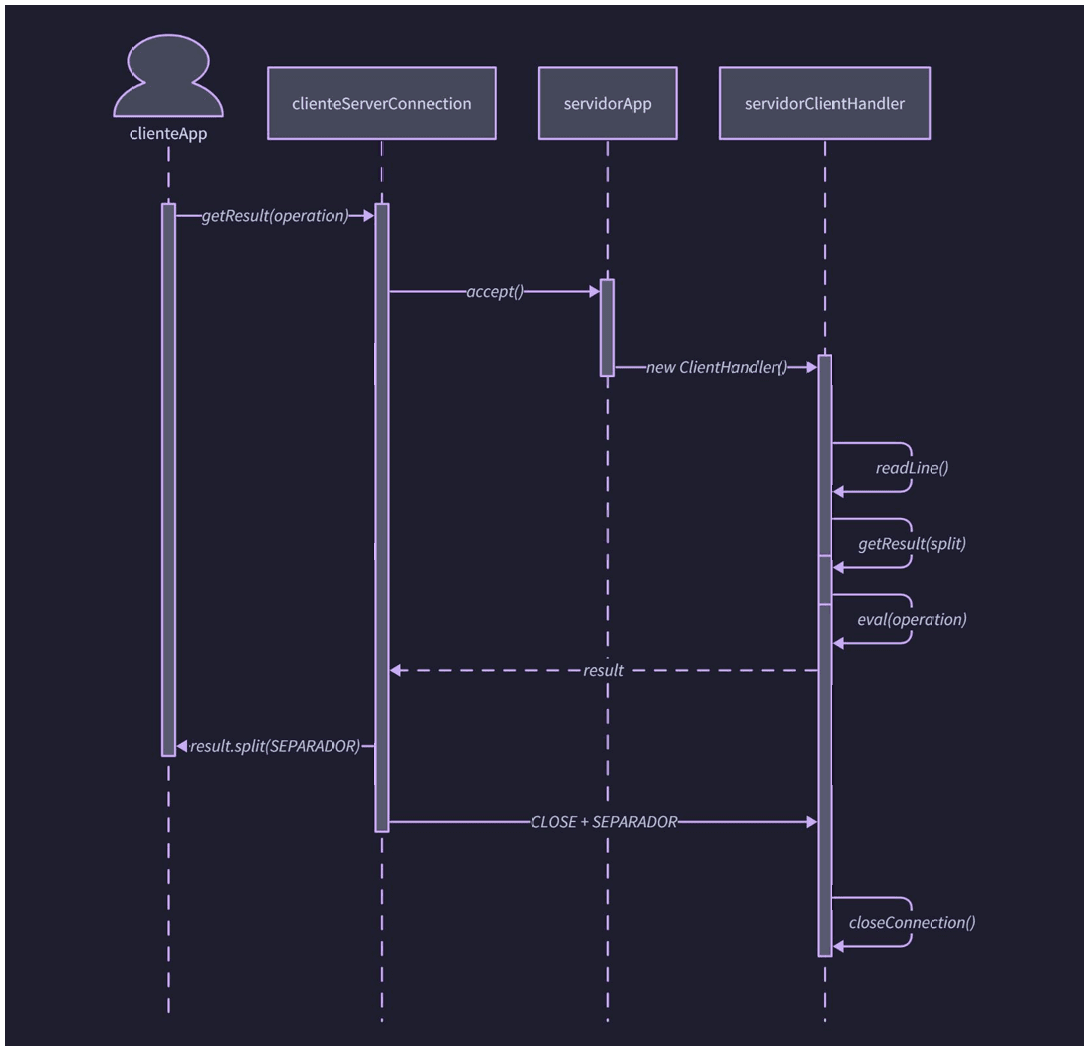
The program is fairly simple, no loops are required, there are no conditions to track, we just have to validate the requests, proccess them in the server and return the responses to be proccessed by the client. However, managing operations based on given strings is challenging and the time constraint didn't leave us more options than rely on an external library for this task, researching the technologies used for this features (script engines) was quite interesting.
Protocol Definition and Use
public enum Protocol {
public final String SEPARADOR = ":";
public final int OPERACION = 0;
public final int RESULTADO = 1;
public final int ERROR = 2;
public final int CLOSE = 3;
}
// Example of request: 0:Math.cos(82)
// Example of response: 1:0.9496776978825432
// Method in the java client that uses the protocol to send requests and read responses
public String getResult(String operation) {
String result = "";
// (...) Some logic for early return in case of input errors
this.out.println("" + OPERACION + SEPARADOR + operation); // Auto-flush is enabled
result = this.in.readLine();
if (result.startsWith("" + ERROR + SEPARADOR)) {
return "ERROR";
}
return result.split(SEPARADOR)[1];
// (...) Exception handling logic
}
Challenges and Solutions
- Challenge: Cross-platform compatibility between Java and C# clients. Solution: Used character-based streams instead of DataInput/OutputStream for compatibility.
- Challenge: Implementing complex mathematical operations. Solution: Integrated JavaScript execution capabilities using the Nashorn library.
- Challenge: Managing multiple client connections. Solution: Implemented multi-threading on the server side to handle concurrent client requests.
Learning Outcomes
- We gained experience in designing and implementing network protocols.
- A better understanding of multi-threaded server applications.
- Learned to integrate different programming languages (Java, C#, JavaScript) in a single project.
- Enhanced error handling and robustness in networked applications.
Unintended Behaviors
It met some mandatory requirements like clients not starting up unless the server is running, however you can see in the recorded demo a little bug once the server is closed, it seems that we should send a server closed signal to empty the buffers and generate errors correctly once the server is closed.
Conclusion
While this project was much shorter than others like the POS manager, it's a good introduction to distributed programming and it's a topic that I hope I can keep working on in the future. And as a footnote; it's true that the java server and client are bundled in the same project, but this was just to speed up the development they can be easily isolated in their own projects.
Friction Coefficient Simulation Project
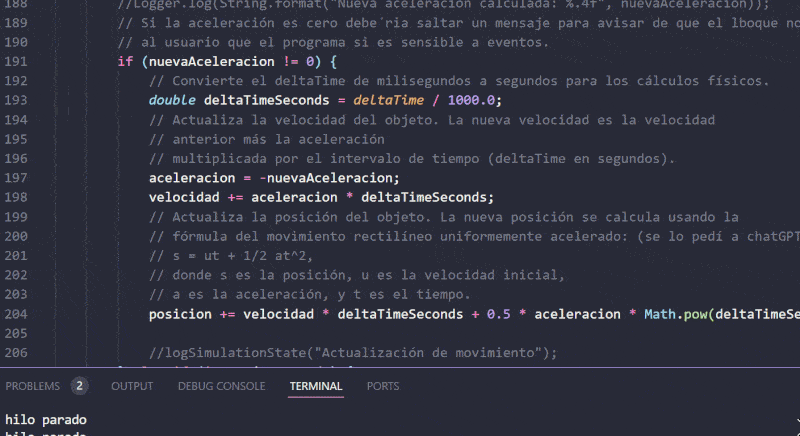
Project Overview
This Java application simulates the movement of an object on an inclined plane, it takes in account various physical properties such as mass, angle of inclination, and the coefficient of friction between different materials. The project also features a visualization with a graphical user interface, allowing users to watch the simulation and adjust different parameters.
Technical Highlights
- Physics Simulation: Implements basic physics calculations to simulate object movement on an inclined plane.
- Java Swing Library: Used to create an interactive user interface.
- Multi-threading: Uses separate threads for animation and GUI updates.
- Custom Painting: Implements custom painting routines for a smooth visualization.
- Material Properties: Incorporates different material properties affecting friction.
- MVC Architecture: Follows Model-View-Controller pattern for better code organization and testing.
Key Features
- Real-time animation of an object sliding on an inclined plane.
- User-adjustable parameters:
- Mass of the object
- Angle of inclination
- Materials for the object and the plane
- Visual representation of different materials using colors and textures.
- Calculation and display of acceleration based on physics principles.
Implementation Details
Physics Calculation
The core of the simulation lies in calculating the acceleration of the object based on the forces acting on it:
private double calcularAceleracion() {
double componenteGravedad = GRAVEDAD * Math.sin(radianesPlano);
double componenteNormal = GRAVEDAD * Math.cos(radianesPlano);
double fuerzaFriccion = coeficienteFriccion * masa * componenteNormal;
double aceleracion = componenteGravedad - (fuerzaFriccion / masa);
return aceleracion > 0 ? aceleracion : 0;
}
This method takes into account the angle of inclination, gravity, and the friction force to determine the object's acceleration.
Animation Loop
The animation is handled in a separate thread using a Timer:
class ActualizarTarea extends TimerTask {
public void run() {
if (fin || estaFueraDeLaVentana()) {
timer.cancel();
timer.purge();
fin = true;
System.out.println("hilo parado");
return;
}
actualizarMovimiento(DELTA_TIME);
actualizarPosicionPeso();
panel.repaint();
}
}
This ensures smooth animation while keeping the UI responsive.
Material Representation
The abstract class Material
allow us to use the principle of dependency inversion:
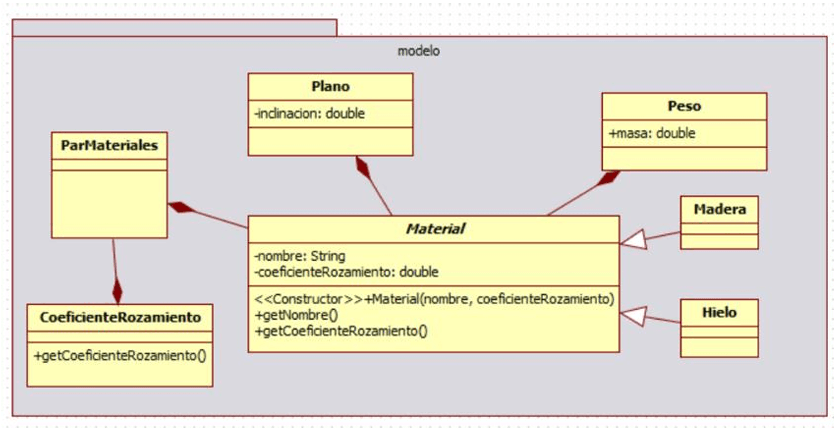
This approach allows for easy addition of new materials and their visual representations
Challenges and Solutions
- Challenge: Implementing realistic physics in a simple simulation. Solution: Carefully researched and implemented basic physics formulas for inclined plane motion and friction.
- Challenge: Creating a responsive UI while running continuous animation. Solution: Use of multi-threading to separate the animation logic from the UI thread.
- Challenge: Representing different materials visually. Solution: Implemented custom painting routines using Java 2D graphics to create unique textures and gradients for each material.
Learning Outcomes
- Gained practical experience in applying physics concepts in software development.
- Improved skills in Java Swing GUI development and custom graphics rendering.
- Enhanced understanding of multi-threaded programming in Java.
Conclusion
While this simulation is far from perfect (for example only the dynamic friction coefficient is accounted), it ignited my interest in Math, to see them being applied in a practical enviroment encourages me to study them again and see what else I can do with the knowledge gained.
Other Highlights
There are other projects that I think are worth showcasing, but to keep things more or less brief I will just show you the demos and point out a couple of features or points of interest:
Juanito Brokers SL (Stock Market Client-Server App)
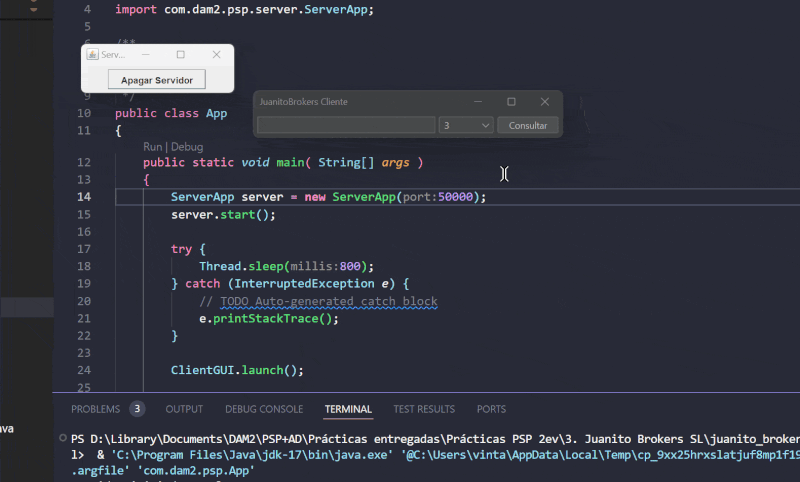
- Server implements multi-threading to handle multiple clients.
- Database management and persistence: Server implements a connection to an Access database.
- DAO pattern: Separates the business logic from the data access logic.
- Real data visualization: The application uses real data fetched from Yahoo Finances, this data is cleaned up using a Python script, which then is imported into the database. The visualization is handled in the client using the
org.knowm.xchart
library.
App for Weather Forecast API
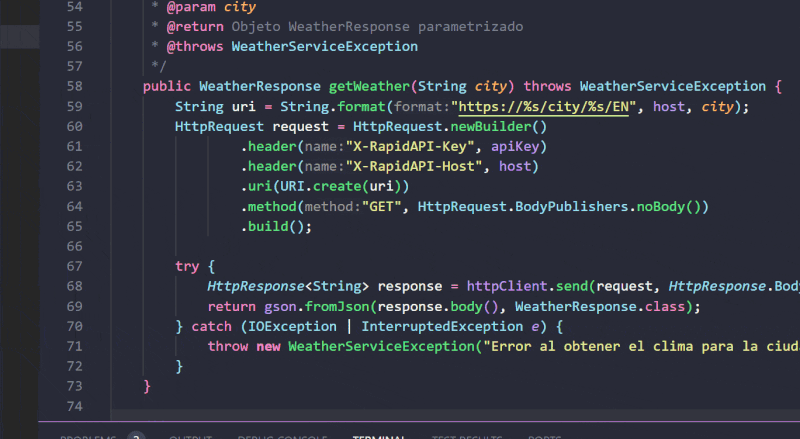
- Api Integration: Successful integration with a thrid-party API (OpenWeather), using java.net.http and google.gson libraries.
- Error Handling and User Experience: Robust error handling provides clear feedback to users when issues occur (invalid city names or API errors).
- Temperature Conversion: Utility classes handle the conversion between Fahrenheit and Celsius.
- Configuration Management: This project implements best practises and enhanced security by using an application.properties file protecting sensitve information like API keys.
Kotlin Note Taking App
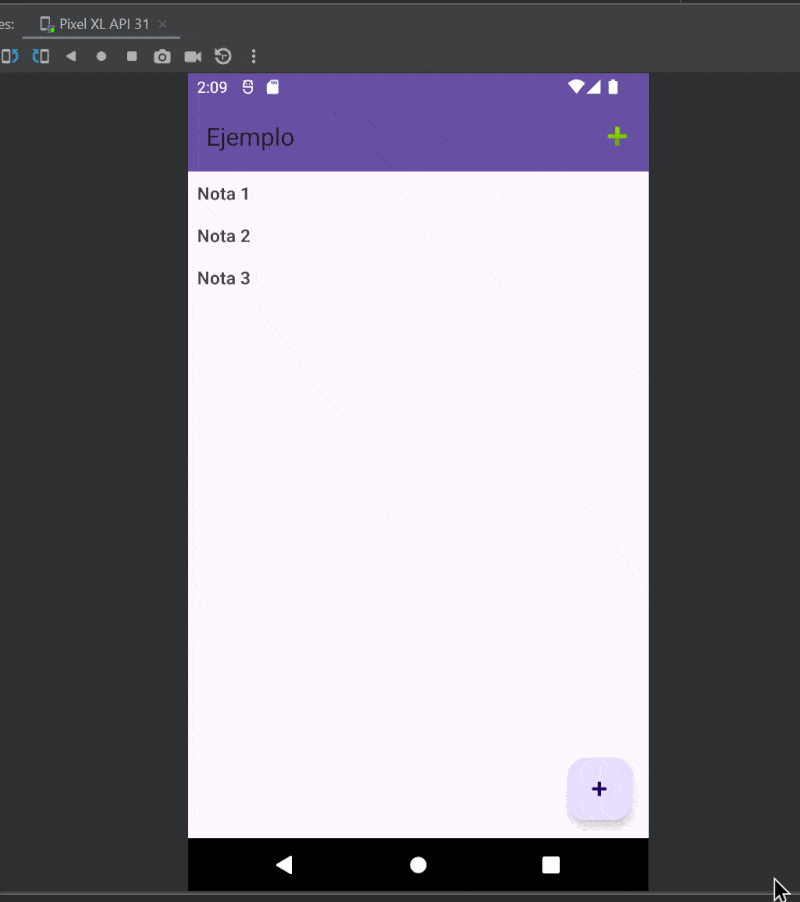
While this app is fairly simple, it showcases important concepts of Android development:
- Kotlin: Nowadays the proferred language for Android development.
- Android Jetpack Components: The use of elements such as RecyclerView and ConstraintLayout demonstrates familiarity with modern Android development practices.
- Activity Lifecycle Management: Activity lifecycle events are handled appropriately, particularly in the communication between MainActivity and EditNoteActivity.
- View Binding: The project uses view binding, a feature that generates a binding class for each XML layout file, which is safer and more efficient than findViewById().
I hope you found this writing engagin and easy to read, hopefully you will understand what the Spanish vocational training "Desarrollo de Aplicaciones Multiplataforma" encompass, I don't intent to showcase everything it's learned with this article, and I have to mention that part of the content depends on the criteria of each professor and the circumstances of the class. Besides, I focused this writing on the content of the second year. However, much of what is learned in the first year is, in one way or another, included in these projects.
PS: Repo is empty, I need sometime to organize and upload the projects, look forward it!